我们在写web项目的时候,如果服务器代码出现错误,那么就会产生异常,如果我们没有对这个异常进行处理,那么该异常会在前端页面,也就是浏览器的页面中展示出来,如下图:
这样子对用户来说是非常不友好的。我们可以通过自定义的处理异常组件来进行对这个异常处理,然后将友好的处理结果在前端页面中展示给用户看。下面我们开始自定义实现SpringMVC中的异常处理。
1.SpringMVC中异常处理的原理
在SpringMVC中,其默认的异常处理是逐级向上抛出异常,下面我们开始使用三张图进行理解:
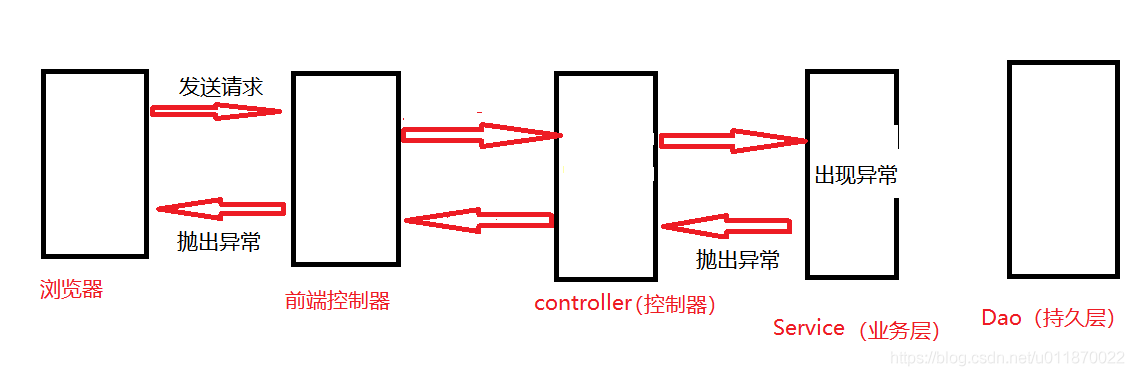
我们要想对这些异常进行处理,SpringMVC在前端控制器提供了一些异常处理组件专门负责处理这些异常,比如:
我们要想实现自定义的异常,需要实现HandlerExceptionResolver中的接口,然后再将我们自定义的异常解析类注册到前端控制器的异常处理组件中。
2.项目的搭建。
2.1新建一个maven的webapp项目。
2.2在pom.xml中引入依赖文件
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>org.example</groupId>
<artifactId>springmvc_exception</artifactId>
<version>1.0-SNAPSHOT</version>
<packaging>war</packaging>
<name>springmvc_exception Maven Webapp</name>
<!-- FIXME change it to the project's website -->
<url>http://www.example.com</url>
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
<maven.compiler.source>1.8</maven.compiler.source>
<maven.compiler.target>1.8</maven.compiler.target>
<spring.version>5.2.8.RELEASE</spring.version>
</properties>
<dependencies>
<!-- https://mvnrepository.com/artifact/org.springframework/spring-context -->
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-context</artifactId>
<version>${spring.version}</version>
</dependency>
<!-- https://mvnrepository.com/artifact/org.springframework/spring-web -->
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-web</artifactId>
<version>${spring.version}</version>
</dependency>
<!-- https://mvnrepository.com/artifact/org.springframework/spring-webmvc -->
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-webmvc</artifactId>
<version>${spring.version}</version>
</dependency>
<!-- https://mvnrepository.com/artifact/javax.servlet/javax.servlet-api -->
<dependency>
<groupId>javax.servlet</groupId>
<artifactId>javax.servlet-api</artifactId>
<version>4.0.1</version>
<scope>provided</scope>
</dependency>
<!-- https://mvnrepository.com/artifact/javax.servlet.jsp/jsp-api -->
<dependency>
<groupId>javax.servlet.jsp</groupId>
<artifactId>jsp-api</artifactId>
<version>2.2.1-b03</version>
<scope>provided</scope>
</dependency>
</dependencies>
<build>
<finalName>springmvc_exception</finalName>
<pluginManagement><!-- lock down plugins versions to avoid using Maven defaults (may be moved to parent pom) -->
<plugins>
<plugin>
<artifactId>maven-clean-plugin</artifactId>
<version>3.1.0</version>
</plugin>
<!-- see http://maven.apache.org/ref/current/maven-core/default-bindings.html#Plugin_bindings_for_war_packaging -->
<plugin>
<artifactId>maven-resources-plugin</artifactId>
<version>3.0.2</version>
</plugin>
<plugin>
<artifactId>maven-compiler-plugin</artifactId>
<version>3.8.0</version>
</plugin>
<plugin>
<artifactId>maven-surefire-plugin</artifactId>
<version>2.22.1</version>
</plugin>
<plugin>
<artifactId>maven-war-plugin</artifactId>
<version>3.2.2</version>
</plugin>
<plugin>
<artifactId>maven-install-plugin</artifactId>
<version>2.5.2</version>
</plugin>
<plugin>
<artifactId>maven-deploy-plugin</artifactId>
<version>2.8.2</version>
</plugin>
</plugins>
</pluginManagement>
</build>
</project>
2.3自定义异常类
package exception;
import org.springframework.web.servlet.HandlerExceptionResolver;
import org.springframework.web.servlet.ModelAndView;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
/**
* Classname:AccountExceptoin
*
* @description:
* @author: 陌意随影
* @Date: 2020-08-08 17:06
* @Version: 1.0
**/
public class AccountException extends Exception {
private String msg;
public AccountException(String msg) {
this.msg = msg;
}
public String getMsg() {
return msg;
}
public void setMsg(String msg) {
this.msg = msg;
}
}
2.4自定义异常解析器,实现AccountExceptionResolver 接口中的方法
package exception;
import org.springframework.web.servlet.HandlerExceptionResolver;
import org.springframework.web.servlet.ModelAndView;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
/**
* Classname:AccountController
*
* @description:
* @author: 陌意随影
* @Date: 2020-08-08 17:05
* @Version: 1.0
**/
public class AccountExceptionResolver implements HandlerExceptionResolver {
@Override
public ModelAndView resolveException(HttpServletRequest httpServletRequest, HttpServletResponse httpServletResponse, Object o, Exception e) {
AccountException accountException = null;
if (e instanceof AccountException){
//强转异常
accountException = (AccountException) e;
}else{
accountException = new AccountException("正在出故障中。。。。。。");
}
ModelAndView modelAndView = new ModelAndView();
System.out.println(accountException.getMsg());
//设置异常信息
modelAndView.addObject("msg",accountException.getMsg());
//设置异常跳转页面
modelAndView.setViewName("erro");
return modelAndView;
}
}
2.5书写控制器代码
package controller;
import exception.AccountException;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
/**
* Classname:AccountController
*
* @description:
* @author: 陌意随影
* @Date: 2020-08-08 18:18
* @Version: 1.0
**/
@Controller
@RequestMapping("/account")
public class AccountController {
@RequestMapping("/testException")
public String testException() throws AccountException {
try {
//模拟异常
// int i=9/0;
}catch (Exception e) {
e.printStackTrace();
throw new AccountException("测试异常页面出错了。。。");
}
return "sucess";
}
}
2.6书写前端代码
2.6.1 错误页面erro.jsp
<%--
Created by IntelliJ IDEA.
User: 陌意随影
Date: 2020/8/8 0008
Time: 17:27
To change this template use File | Settings | File Templates.
--%>
<%@ page contentType="text/html;charset=UTF-8" language="java" isELIgnored="false" %>
<html>
<head>
<title>页面访问错误</title>
</head>
<body>
<h3>${msg}</h3>
</body>
</html>
2.6.2成功页面sucess.jsp
<%--
Created by IntelliJ IDEA.
User: 陌意随影
Date: 2020/8/8 0008
Time: 19:47
To change this template use File | Settings | File Templates.
--%>
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<html>
<head>
<title>成功页面</title>
</head>
<body>
<h2>访问成功</h2>
</body>
</html>
3.6.3测试页面index.jsp
<%--
Created by IntelliJ IDEA.
User: 陌意随影
Date: 2020/8/8 0008
Time: 18:17
To change this template use File | Settings | File Templates.
--%>
<%@ page contentType="text/html;charset=UTF-8" language="java" isELIgnored="false" %>
<html>
<head>
<title>首页</title>
<script>
function btnClick() {
window.location.href="account/testException";
};
</script>
</head>
<body>
<input type="button" id="btn" value="测试异常" onclick=" btnClick()">
</body>
</html>
3.配置文件
3.1在springMvcConfig.xml的配置
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:mvc="http://www.springframework.org/schema/mvc"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="
http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context.xsd
http://www.springframework.org/schema/mvc
http://www.springframework.org/schema/mvc/spring-mvc.xsd">
<!-- 开启注解扫描包-->
<context:component-scan base-package="controller"></context:component-scan>
<!-- <context:component-scan base-package="exception"></context:component-scan>-->
<!-- 配置视图解析器-->
<bean id="internalResourceViewResolver" class="org.springframework.web.servlet.view.InternalResourceViewResolver">
<property name="prefix" value="/WEB-INF/pages/"></property>
<property name="suffix" value=".jsp"></property>
</bean>
<!--开启MVC注解支持-->
<mvc:annotation-driven enable-matrix-variables="true"/>
<!-- 配置自定义异常解析器-->
<bean id="exceptionResolver" class="exception.AccountExceptionResolver"></bean>
</beans>
3.2配置web.xml
<!DOCTYPE web-app PUBLIC
"-//Sun Microsystems, Inc.//DTD Web Application 2.3//EN"
"http://java.sun.com/dtd/web-app_2_3.dtd" >
<web-app>
<display-name>Archetype Created Web Application</display-name>
<!-- 配置前端控制器-->
<servlet>
<servlet-name>dispatcherServlet</servlet-name>
<servlet-class>org.springframework.web.servlet.DispatcherServlet</servlet-class>
<!-- 配置初始化参数-->
<init-param>
<param-name>contextConfigLocation</param-name>
<param-value>classpath:springMvcConfig.xml</param-value>
</init-param>
<load-on-startup>1</load-on-startup>
</servlet>
<servlet-mapping>
<servlet-name>dispatcherServlet</servlet-name>
<url-pattern>/</url-pattern>
</servlet-mapping>
<!-- 配置字符集编码过滤器-->
<filter>
<filter-name>characterEncodingFilter</filter-name>
<filter-class>org.springframework.web.filter.CharacterEncodingFilter</filter-class>
<init-param>
<param-name>encoding</param-name>
<param-value>UTF-8</param-value>
</init-param>
</filter>
<filter-mapping>
<filter-name>characterEncodingFilter</filter-name>
<url-pattern>/*</url-pattern>
</filter-mapping>
</web-app>
4.开始测试
4.1测试没有异常时
4.2去掉注释,模拟测试异常
5.此次进行测试异常是在控制器controller层面出现异常,在其它地方处理异常也是一样的。此次测试代码已经上传到个人博客,如有需要自行下载解压后以maven工程导入:http://moyisuiying.com/wp-content/uploads/2020/08/springmvc_exception.rar